Problem:
The gray code is a binary numeral system where two successive values differ in only one bit.
Given a non-negative integer n representing the total number of bits in the code, find the sequence of gray code. A gray code sequence must begin with 0 and with cover all 2n integers.
Have you met this question in a real interview? Yes
Example
Given n = 2, return [0,1,3,2]. Its gray code sequence is:
00 - 0
01 - 1
11 - 3
10 - 2
Note
For a given n, a gray code sequence is not uniquely defined.
[0,2,3,1] is also a valid gray code sequence according to the above definition.
Challenge
O(2n) time.
Solution:
Use recursion to generate a new gray code from the (n-1) as the picture shown in the Figure below.
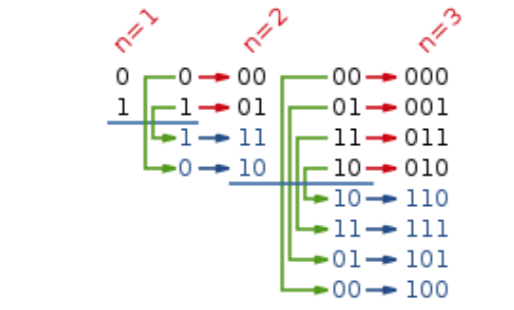
/*************************************************************************
> File Name: GrayCode.java
> Author: Yao Zhang
> Mail: psyyz10@163.com
> Created Time: Fri 13 Nov 17:22:56 2015
************************************************************************/
public class GrayCode{
List<Integer> grayCode(int n){
ArrayList<Integer> result = new ArrayList<Integer>();
result.add(0);
if (n == 0)
return result;
if (n == 1){
result.add(1);
return result;
}
result = (ArrayList<Integer>) grayCode(n - 1);
ArrayList<Integer> temp = reverse(result);
for (int i = 0; i < temp.size(); i++){
temp.set(i,(1 << (n - 1)) + temp.get(i));
}
result.addAll(temp);
return result;
}
public ArrayList<Integer> reverse(ArrayList<Integer> result){
ArrayList<Integer> newResult = new ArrayList<Integer>();
for (int i = result.size() - 1; i >= 0; i--){
newResult.add(result.get(i));
}
return newResult;
}
}